Overview The universal asynchronous transceiver (UART) or serial port is the standard peripheral for most microprocessors or microcontrollers. The asynchronous serial port provides a simple way for communication between the two microcontrollers (or between the microcontroller and the serial device of the slave) without requiring the same system clock. With the appropriate level converter, the serial port can also communicate with RS-232 and RS-485 networks, or connect with the COM port of a PC. The serial port connection only requires 2 signal lines (Rx and Tx) and can achieve full-duplex communication. As long as the devices at both ends of the communication use the same data bit format and baud rate, data can be successfully transmitted without any other information between the devices .
This application note introduces a general 10-bit asynchronous serial port solution using software. The design example uses a low-power MAXQ3210 microcontroller, which does not include a hardware serial port. When sufficient hardware serial ports cannot be provided in specific applications, the same method can be used to add one or more serial ports to any MAXQ® microcontroller, such as the MAXQ2000.
The advantage of software UART Some people may ask: At present, most microcontrollers have powerful hardware serial ports, why do you still use software port of microcontroller to realize software UART? The main reasons are as follows:
Although most microcontrollers have a hardware serial port, some microcontrollers do not have a UART hardware serial port. When choosing a microcontroller for a system, it is difficult to find a perfect solution that meets all requirements. Therefore, by adding the software design of the microcontroller to realize certain peripheral functions can make up for the "defects" of the microcontroller's function and improve the design flexibility.
Some microcontrollers have a hardware UART, but for some reason, it may not be able to meet specific design requirements. For example, the microcontroller needs to communicate with a peripheral that works on a slightly different serial protocol, or the number of data bits, parity check, and input / output buffers supported by the hardware UART do not fully meet the application requirements. Building software UART can provide greater flexibility in the definition of UART function and the details of serial port protocol.
The microcontroller may have a hardware UART that is very suitable for the application requirements, but the number is not enough. At this time, in addition to adding a chip to increase the number of serial ports, you can also simply add a software UART that is consistent with the microcontroller UART function to meet system requirements.
How much bandwidth the software UART will occupy the main program is also very important. The main reason for using the hardware UART (or other serial communication peripherals) is that the microcontroller does not have to spend time dealing with the underlying protocol of serial communication. The lengthy data bit sampling, time slot counting, and input / output shift are all handled by hardware. The UART sends an indication signal (interrupt or other indication flag) to the main microcontroller to indicate that it has received a character or completed a transmission task. The microcontroller can quickly load or unload the data in the UART buffer area as needed, and then return to handle its core tasks.
In short, the software UART means that the program needs to spend more time observing the serial communication of the port pins, and some designs cannot accept this time-consuming operation.
Fortunately, we can avoid excessively occupying the time and resources of the microprocessor when designing the software UART. Below we consider the process of receiving and sending a character, assuming the use of the standard 10-bit asynchronous serial protocol (1 start) Bits, 1 stop bit, and 8 data bits) (Figure 1).
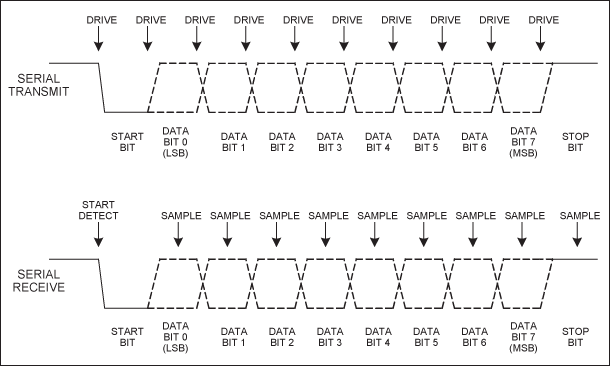
Figure 1. 10-bit asynchronous serial communication protocol transmission and reception
Once the transmit or receive operation is started, the serial UART (whether in software or hardware) does not need to continuously monitor the I / O lines. When sending a character, the UART only needs to drive the state of the transmission line once per data bit period, and establish the level of each data bit in turn, from the start bit to the data bit to the stop bit. When receiving characters, the UART starts at the first falling edge. After the falling edge, the UART only needs to sample the state of the transmission line at the middle of each time slot.
Function of software UART We can describe the function of software UART as a pair of state machines: one for sending characters and the other for receiving characters. For a full-duplex UART, the two state machines work in parallel and require two independent timed interrupts. The two state machines have a start mode and a stop mode. The sending state machine exits its idle state for data transmission when a character is sent, and returns to its idle state after sending the stop bit. The receiving state machine exits its idle state and starts receiving data when it detects a falling edge on the receiving line. Once the low-level start state is detected (indicating that the start bit has started), it starts counting the data bit slots and samples the data line as needed, including the stop bit.
In order to avoid taking up too much unnecessary main program time, the UART state machine should be triggered periodically by a timed interrupt. Initialized falling edge detection for data reception is handled separately by external edge-triggered interrupts. If the timer of the state machine is set to start an interrupt at each data bit, the state machine can perform any required operation each time the interrupt is triggered (the next state can be entered if necessary). The state machine code should be optimized as much as possible, because the software UART will continuously run these codes in the background.
Designing with MAXQ3210 In order to understand the processor resources required by the UART function, as an example, we discuss the software UART implemented with Maxim's MAXQ3120. The microcontroller uses a 28-pin package, 5V power supply, can run at about 3.57MIPS, contains 15 port pins, but no internal UART. The MAXQ3210 also includes other functions suitable for our design requirements: a periodic timer that provides interrupts for the transmit and receive state machines; and an external interrupt that is used to detect the falling edge of the received data line. Since the MAXQ3210 has only one periodic timer, the software UART will use half-duplex mode, which means that it can only send or receive data at a certain moment, and cannot receive and send at the same time. In fact, this is not a problem for most communication and control protocols.
For the MAXQ3210, receiving and sending state machines and subroutines for setting modes, loading and unloading characters can be implemented with 171 instruction words. For example, the sending state machine has only three states (data bit, stop bit, and return to idle), as shown below:
intTX_bit: move GRH, PSF move GRL, AP move T2CNB.3, # 0; Clear TImer 2 overflow flag move AP, # 5 rrc; Start with least significant bit jump C, intTX_bit_one intTX_bit_zer move TXDO, # 0 jump intTX_bit_next intTX_bit_one: move TXDO, # 1 jump intTX_bit_next intTX_bit_next: djnz LC [1], intTX_bit_done move IV, #intTX_stop intTX_bit_done: move AP, GRL move PSF, GRH reTI intTX_stop: move T2CNB.3, # 0; Clear TImer 2 overflow flag move TXDO, # 1; Float high move IV, #intTX_idle reTI intTX_idle: move A [4], #SER_MODE_TX_IDLE move T2CNB.3, # 0; Clear timer 2 overflow flag move T2CNA.7, # 0; Disable timer 2 interrupts move T2CNA.3, # 0; Stop timer reti Download (ZIP, 5.6kB) the complete code of this application note.
In any case, the interrupt code occupies a maximum of 19 instruction cycles, and the worst-case bandwidth shown in Table 1 can be estimated based on the baud rate. Note: The worst-case bandwidth is estimated based on the operation of continuously sending or receiving characters. Once the character transmission is completed, the UART will be turned off, allowing other operations of the main program before starting to transmit the next character.
Table 1. Bandwidth occupied by MAXQ3210 for UART communication
Baud Rate | Cycles per Timer Tick (at 3.57MHz) | Worst-Case Interrupt Cycle Count | Bandwidth Used by UART (%) |
9600 | 372 | 19 | 5 |
19200 | 186 | 19 | 10 |
28800 | 124 | 19 | 15 |
57600 | 62 | 19 | 31 |
Conclusion This application note introduces a simple solution that uses software to implement 10-bit asynchronous UART communication through 2 standard ports. As long as the microcontroller's free port pins can meet the needs, the above solutions can also be used to implement SPI ™ to SMBus ™ Any serial communication of I²C.
Here, we use the relatively low-speed MAXQ3210 microcontroller for testing. If you use a high-speed microcontroller (such as MAXQ2000 or high-speed 8051), you can implement more than one software UART, which can be configured to half duplex or full duplex Working mode (or more complicated serial communication peripherals), and will not occupy more main program bandwidth. When software is used to communicate with peripherals, any part of the protocol can be modified to maintain consistency with other UART devices or related standard changes, providing maximum flexibility for new designs.
A similar article was published in the online version of Embedded Systems Design, February 2007.
Led Ball String Light
Best decoration for Christmas tree, outdoor trees, porch, around the patio table umbrella, Xmas, wedding, birthday party, outside, indoor.
Each string lights has a socket that can be connected to another string lights for infinite extension .Fairy lights with soft wire is very flexible to hang in kids bedroom,tent,etc.
Waterproof IP67, Non-friable ,not overheat, high voltage protection, cool to the touch.
The bulbs are each one color a piece, which I didn't know when I bought this, but I'm alright with that. They're cute, the frosted white globe over the LED bulb is nice. The colors, in order, are Blue, Orange (or dark yellow), Green, and Red, like you might find on traditional multicolor lights. The picture looks like there's purple or teal lights, but that's not true. The remote came with a battery, which was cool. The length stretches over my entire porch, exactly, which was a great surprise.
Led Ball String Light
Led String Lights Outdoor,Cotton Ball String Lights,Cotton Ball Fairy Lights,Cotton Ball Fairy Lights
XINGYONG XMAS OPTICAL (DONGGUAN ) CO., LTD , https://www.xingyongxmas.com